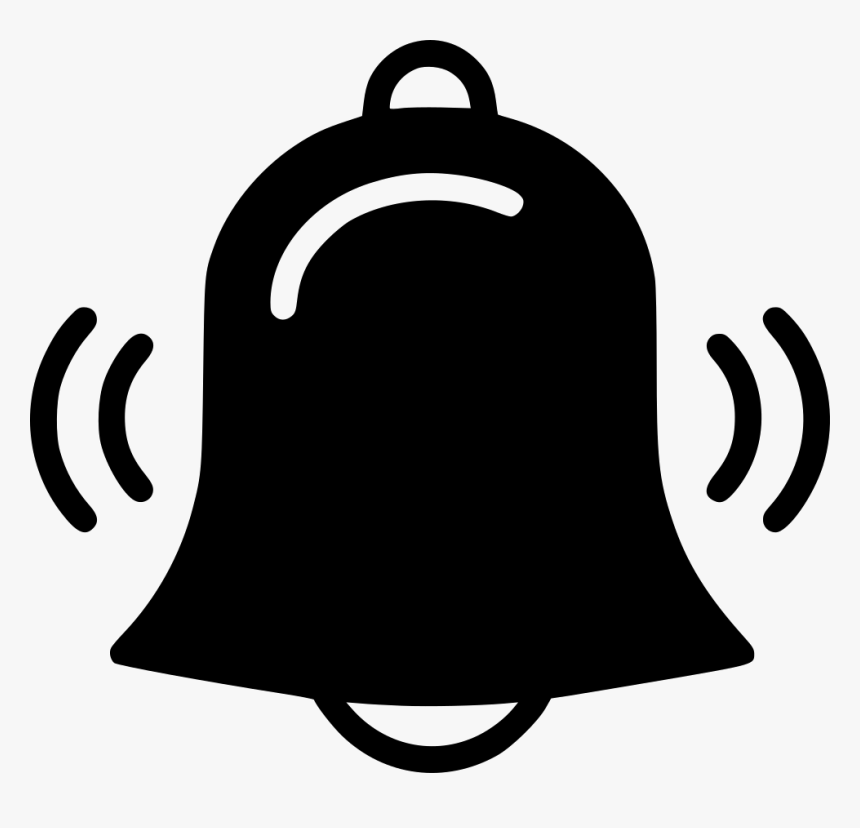
The Notifications API allows web applications to send push notifications to users similar to native OS applications. This means web apps can notify users even if the application is idle or in the background.
TL;DR: CodeSandBox Link
Compatibility Disclaimer: IE 11 and Safari on iOS don't support Notifications API. Every modern desktop browser does though, and you can check out the compatibility table over at MDN.
Step 1: Get User Permission
Your website must request the user's permission to send notifications. The Notifications API won't work without it. Additionally, your website must be in a secure context, i.e., using https or Chrome and Firefox will not let the request through.
For obvious anti-spam and UX reasons, triggering notifications without a user action (like button click) will be forbidden in most browsers going forward.
Recap:
- your website must get user's permission to send notifications
- which will only go through if your website is using https
- and it must be triggered on a user action such as click, and not whenever page loads
With that out of the way, using the Notifications API to rquest permission is straightforward:
// Using the promise-based Notification.requestPermission method:
Notification.requestPermission().then(function(result) {
// do something with result
});
Step 2: Handle User Permission Response
After the user has responded to the permission request, you need to handle it in your code. This handler needs to be called inside the .then()
on the Notification.requestPermission()
call as shown above.
Like this:
Notification.requestPermission()
.then(permission => handlePermission(permission));
In your handler, check if the permission has been granted by checking Notification.permission
. If it's 'denied' or 'default', then you can't do much as the user refused permission. If it's 'granted', you can now create notifications!
Here's my handlePermission
function:
const handlePermission = permission => {
// Whatever the user answers,
// we make sure Chrome stores the information
if (!("permission" in Notification)) {
Notification.permission = permission;
}
if (
Notification.permission === "denied" ||
Notification.permission === "default"
) {
// can't show notifications
} else {
// cool we have the user's permission
// we can now create notifications
}
};
Step 3: Create Notification(s)
Assuming the user granted you permission in the previous steps, you can now show them notifications.
Showing Notifications is easy, you simply call the Notification()
constructor with new
and pass it the relevant parameters.
var myNotification = new Notification(title, options);
Title is a String and is required, options is an optional object.
The possible options are: dir, lang, body, tag, icon, image, data, vibrate, renotify, requireInteraction, actions, and silent.
You can read what these are over at MDN
The ones we'll be using are body
and icon
.
const dt = new Date().toLocaleTimeString();
const text = `this is the time right now: ${dt}`;
const notification = new Notification("Check this out!", {
body: text,
icon: "https://uploads.codesandbox.io/uploads/user/c2c4c908-13fc-4dae-a346-b2b8ef148a78/rmzS-react-1-282599.png"
});
Here, I'm passing a string with the local time as the body
option and the url to an img file as the icon
option.
Step 4: Close the notification (Optional)
Finally, in the interest of user experience, you can call the close function on the notification instance, like below.
// close the notification after 3 seconds
setTimeout(notification.close.bind(notification), 3000);
Notice that we need to have a reference to the specific Notification instance in order to close it.