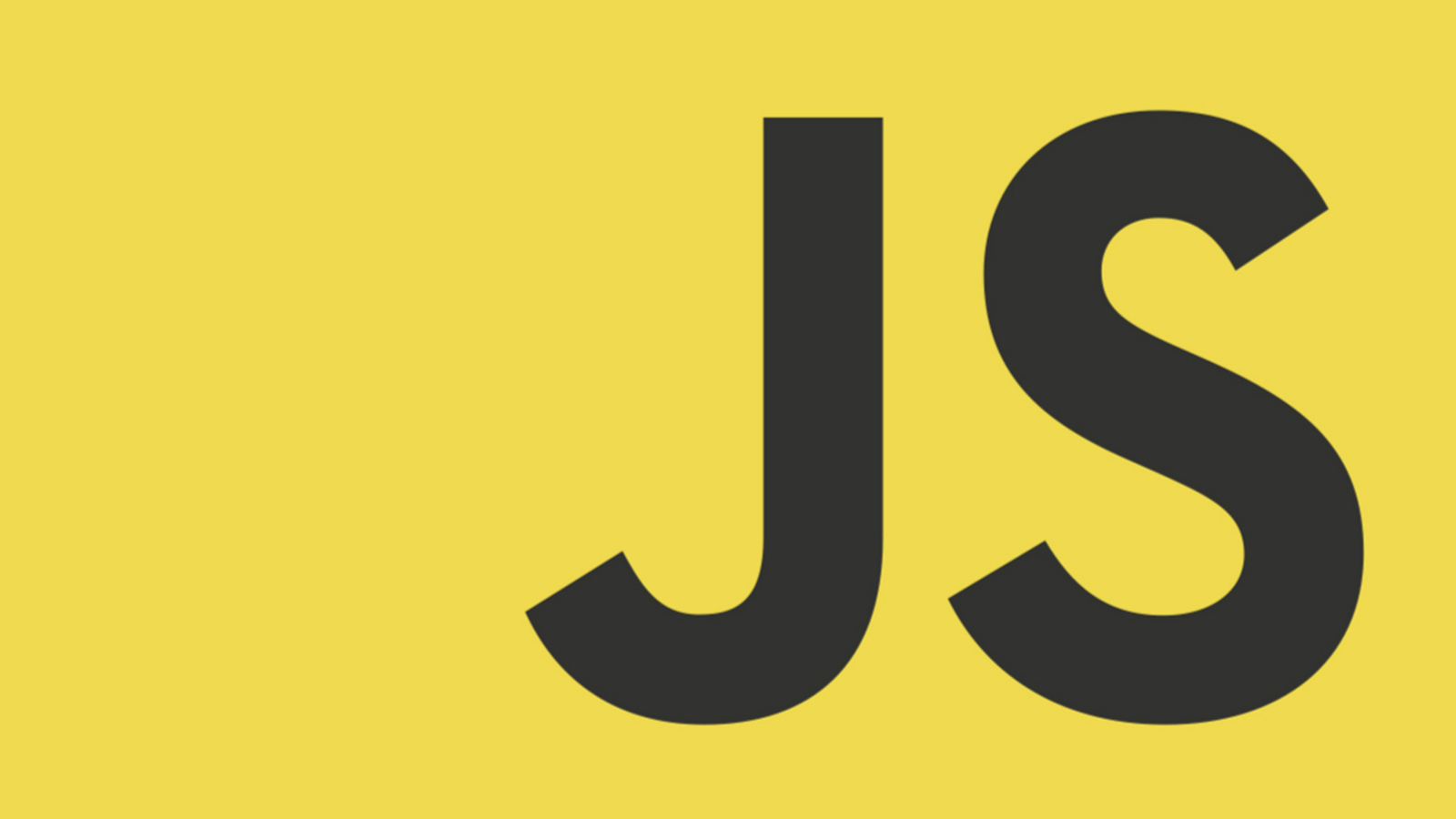
Here are some frequently asked JavaScript interview questions. You can expect to be asked these types of questions in a phone screening or a first round interview, depending on the position and your experience.
What is scope?
scope is the set of rules that determines where and how a variable (identifier) can be looked-up. This look-up may be for the purposes of assigning to the variable, which is an LHS (left-hand-side) reference, or it may be for the purposes of retrieving its value, which is an RHS (right-hand-side) reference.
What is closure?
A closure is the combination of a function bundled together (enclosed) with references to its surrounding state (the lexical environment). In other words, a closure gives you access to an outer function’s scope from an inner function. In JavaScript, closures are created every time a function is created, at function creation time.
In the snippet below, the logName function 'remembers' the name in randomFunc, even when it is called by newFunc.
const randomFunc = () => {
const name = 'Ajaypal';
const logName = () => console.info(name);
return logName;
}
const newFunc = randomFunc();
newFunc();
// logs 'Ajaypal' to the console
What is this
in JavaScript?
this
is a reference to an execution context (global, function or eval) that, in non–strict mode, is always a reference to an object and in strict mode can be any value. In most cases, the value of this
is determined by how a function is called (runtime binding).
this
Determination:
- if the function is called with
new
, the object instance is thethis
- if you set the
this
withbind()
,call()
, orapply()
, the argument you pass in will be thethis
- if the function is called in a containing object (context), that context becomes the
this
- default in non-strict mode: the
Window
in Browsers, the Global Object in Node - default in strict-mode:
undefined
What's the difference between null
and undefined
?
null |
undefined |
---|---|
null is a primitive value, it is NOT a type. | undefined is a type with the value undefined |
null means the variable/reference has no value. | when variable is declared but not assigned a value, it has the value undefined. |
the typeof null is 'object' (which is a famous bug in JS). |
the typeof undefined is 'undefined' |
What is instanceof
useful for?
The instanceof
operator tests whether the prototype property of a constructor appears anywhere in the prototype chain of an object.
Its useful for testing membership of custom types and complex built-in types like RegExp, Dates, Arrays, Objects, etc.
Example:
Custom-types
const ClassFirst = function() {};
const ClassSecond = function() {};
const instance = new ClassFirst();
instance instanceof Object; // true
instance instanceof ClassFirst; // true
instance instanceof ClassSecond; //false
RegExp:
/regularexpression/ instanceof RegExp; // true
Arrays:
[] instanceof Array; // true
Objects:
{} instanceof Object; // true
Dates:
const dt = new Date();
dt instanceof Date; // true
What is typeof
useful for?
The typeof
operator returns a string indicating what the type of an operand is.
Its useful for determining whether something is a primitive type or not, i.e., for finding whether something is a Number, String, Boolean, Undefined, BigInt, Symbol, Function or Object.
String
'example string' instanceof String; // false
typeof 'example string' === 'string'; // true
Number
99.99 instanceof Number; // false
typeof 99.99 === 'number'; // true
Boolean
true instanceof Boolean; // false
typeof true === 'boolean'; // true
Undefined
const b;
typeof b === 'undefined'; // true
Function
const func = () => {};
typeof func === 'function'; // true
Symbol
const sym = Symbol('foo');
typeof sym === 'symbol'; // true
BigInt
const big = BigInt(42);
typeof big === 'bigint'; // true
Object
typeof {} === 'object'; // true
Why is typeof null === 'object'
!?
In the first implementation of JavaScript, JavaScript values were represented as a type tag and a value. The type tag for objects was 0. null was represented as the NULL pointer (0x00 in most platforms). Consequently, null had 0 as type tag, hence the "object" typeof return value.
Source: MDN
Basically, it was implemented like that in the first version of JavaScript and we are stuck with it, since fixing this will break a lot of legacy code.