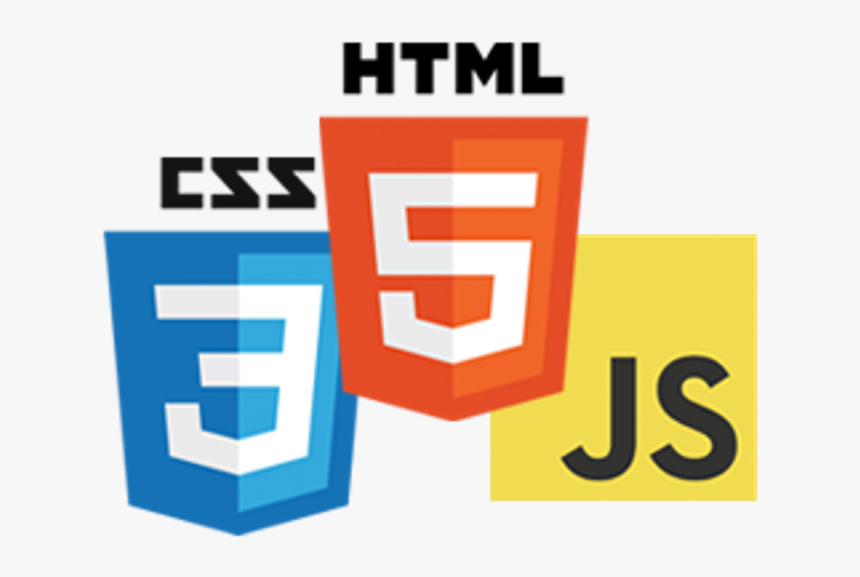
Here's a brain dump of different frontend interview questions from my personal archive. In no particular order.
What are the building blocks of HTML5/WHATWG?
- Semantic markup (article, strong, em, mark, etc)
- ES6/ES2015
- Video and Audio API
- Canvas and SVG
- Geolocation API
- Communication API/Websockets
- Web Worker API
- WebStorage API
Explain your understanding of the box model and how you would tell the browser in CSS to render your layout in different box models.
The box model determines how much space an element will take on the screen.
It includes the height/width (applied or generated), the padding, and the borders.
The paddings and borders are usually set. The height/width is often flexible, and is determined by the inner text or child elements.
Describe Floats and how they work.
Float will “push” an element to the side you’ve chosen, either left or right.
That’s why you don’t have a float: center.
It’s simple to understand and see how it affects the targeted element.
But the issues come from the surroundings:
a parent with only floated childs will have a height of zero, as if the children weren’t there.
The text that follows a floated element will be wrapped around it.
If you have float: left, the text will use the remaining space on the right, and then the one remaining below.
A float will take an element out of the flow, a bit like position absolute or position fixed but not exactly.
The float is directly related to the clear property, which reintroduces the floated element in the flow, by placing a cleared element after the floated one.
What does * { box-sizing: border-box; }
do? What are its advantages?
If you set a width, and add paddings and borders, the total width won’t change. It’s the innerwidth that’ll adapt.
You can play with the paddings and border values without worrying about expanding your box.
Convenient for column layouts. And you can mix percentage and pixel values, so you don’t have to rely on a child element for the padding.
What's the difference between a relative, fixed, absolute and statically positioned element?
position:static
: it is the default value. The element stays in the flow.
position:relative
: also stays in the flow, but you can use left/right/top/bottom
to offset
the element, without the other elements being affected by it. It also allows you to use the z-index. Relatively positioned elements act as a point of reference for absolute-positioned child elements.
position:absolute
: it takes the element out of the flow. So the other elements act like it’s not there. That’s why absolute elements appear on top.
position:fixed
: Fixed positioning is like absolute
, as it takes the element out of the flow but the reference is the body, or the viewport. It's usually used for headers and overlays, because fixed elements don’t scroll.
What's the difference between display:inline
and display:inline-block
?
display:inline
elements can’t have padding-top, padding-bottom, margin-top, margin-bottom.
display:inline-block
elements can have padding-top, padding-bottom, margin-top, margin-bottom.
What's the difference between em
and rem
?
em
is relative to the font-size of its direct or nearest parent.
rem
is only relative to the html (root) font-size.
Can you describe the difference between progressive enhancement and graceful degradation?
Progressive enhancement: serve a basic website compatible with older browsers and enable new functionality for latest browsers using feature-detection.
Graceful degradation: start with swanky website optimized for latest browsers and turn off unsupported functionality for older browsers using feature-detection.
How would you optimize a website's assets/resources?
Minification
Lazy-loading scripts and images
Use CDNs for frameworks and 3rd party libs
Use only what you need from frameworks and 3rd party libs
Remove unnecessary or under-used resources
Use Bundle analyzers and implement code-splitting if needed
Most of this is done for you by different frameworks and tools like NextJS, create-react-app or angular cli, but knwoing the why and how is still relevant.
How many resources will a browser download from a given domain at a time?
Between 2 and 8 depending on browser.
Describe the difference between a cookie, sessionStorage and localStorage.
sessionsStorage and localStorage are part of the WebStorage API, which was introduced with HTML5.
sessionStorage
: stores information as long as the session goes. Usually until the user closes the tab/browser.
localStorage
: stores information as long as the user does not delete them.
cookie
: cookies are simply cookies, which are supported by older browsers and usually are a fallback for frameworks that use the above mentioned WebStorages.
Describe the difference between <script>
, <script async>
and <script defer>
.
<script>
: browser will run your script immediately,
before rendering the elements that are below your script tag.
<script async>
: browser will continue to load the HTML page and render it while the script is loaded and executed asynchronously.
<script defer>
: browser will run your script when the page is finished parsing.The order of scripts is maintained in defer; no guarantees in async.
Why is it generally a good idea to position CSS <link>
tags in the <head>
tag and <script>
tags at the end of the <body>
tag?
You want content to be styled and rendered ASAP, whereas scripts can execute after everything else is rendered.
What is the difference between classes and ID's in CSS?
Use IDs for individual elements.
Use Classes for groups of elements.
IDs have higher priority as selectors.
What's the difference between "resetting" and "normalizing" CSS? Which would you choose, and why?
Use Normalize. It standardizes all the browsers to a set default.
Reset just resets all the defaults.
Describe z-index
and how stacking context is formed.
z-index
is for visual depth. An element needs to be positioned (relative, absolute, fixed) for the z-index to work.
The natural depth depends upon a couple of things:
if an element is nested within another one, then it shows up on top of its parent.
if a sibling comes after another sibling, it’s on top as well.
position:absolute
or position:fixed
will upset this. They’ll show up on top regardless of their tree position.
When elements overlap you can use the z-index property to make sure they will “stack” upon each other.
The z-index value is relative. So an element with z-index:2
will stack on top of element with z-index:1
.
Describe BFC(Block Formatting Context) and how it works.
A block formatting context is a part of a visual CSS rendering of a Web page. It is the region in which the layout of block boxes occurs and in which floats interact with each other.
A block formatting context is created by one of the following:
- the root element or something that contains it
- floats (elements where float is not none)
- absolutely positioned elements (elements with
position: absolute
orposition: fixed
) - inline-blocks (elements with
display: inline-block
) - table cells (elements with
display: table-cell
, which is the default for HTML table cells) - table captions (elements with
display: table-caption
, which is the default for HTML table captions) - elements where overflow has a value other than visible
- flex boxes (elements with
display: flex
ordisplay: inline-flex
)
Explain the following request and response headers:
Expires
: Gives the date/time after which the response is considered stale
Date
: The date and time that the message was sent
Age
: The age the object has been in a proxy cache in seconds
If-Modified-Since
: Allows a 304 Not Modified to be returned if content is unchanged
DNT
(Do Not Track): Requests a web application to disable their tracking of a user.
Cache-control
: Specifies directives that must be obeyed by all caching mechanisms along the request-response chain
Transfer-Encoding
: The form of encoding used to safely transfer the entity to the user.Currently defined methods are:
* chunked
* compress
* deflate
* gzip
* identity
ETag
: An identifier for a specific version of a resource, often a message digest
X-Frame-Options
: Clickjacking protection.
* deny
- no rendering within a frame
* sameorigin
- no rendering if origin mismatch
* allow-from
- allow from specified location
* allowall
- non-standard
* allow
from any location